How to Send Text Messages From IBM i
Communication is often done via email, but a text message can do the trick, too. In fact, you probably already have the tools you need to send a text message installed on your IBM i
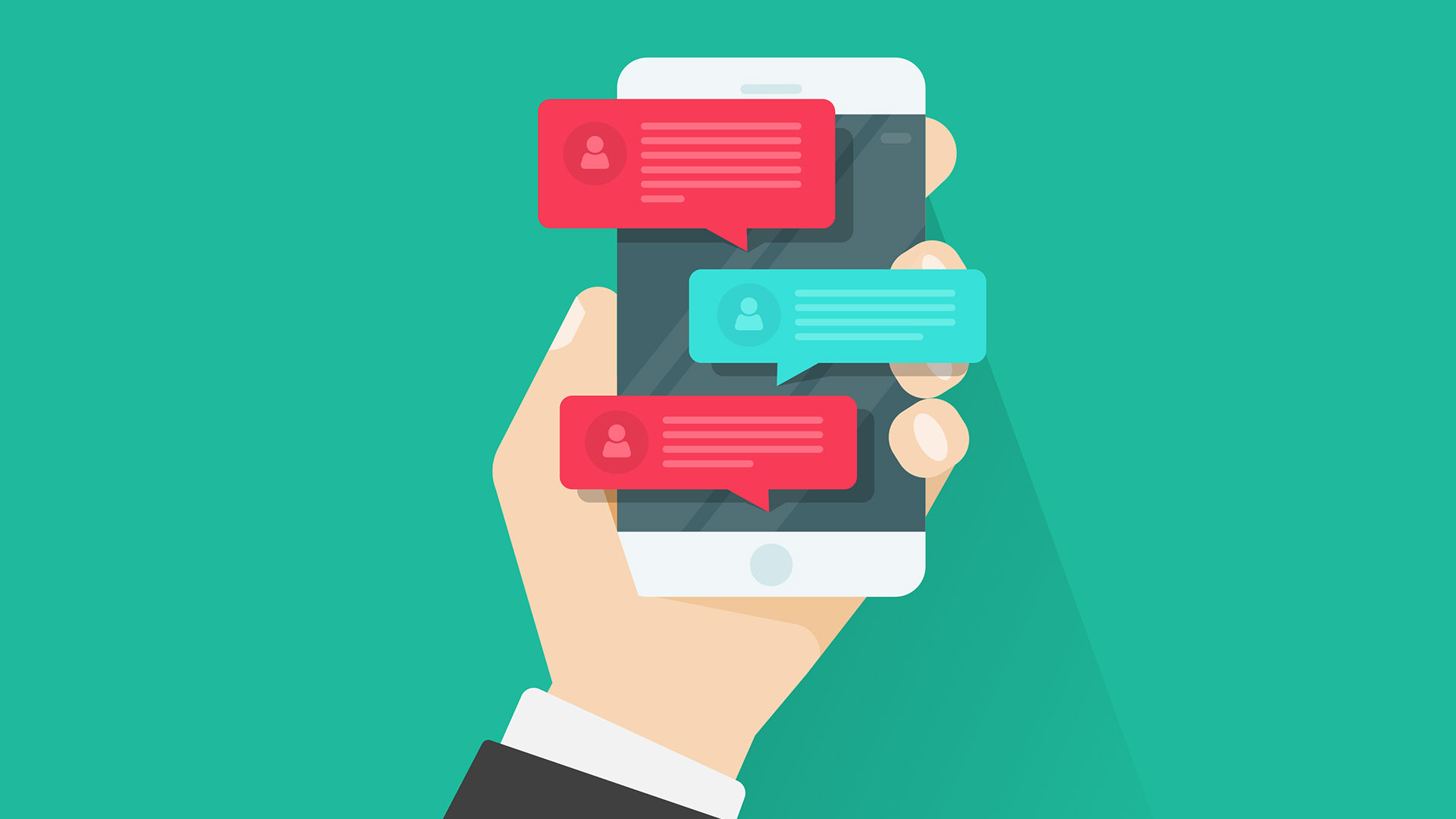
Communication is one of the most critical factors in running a successful business. This could mean informing a customer their order has shipped or letting your IT manager know a process has an issue. You might want to send an invoice or a photo of a product.
Typically, this would be done via email, but have you ever wondered if a text message might do the trick? It can, and it’s a lot easier than you’d imagine. In fact, you probably already have the tools you need installed on your IBM i. What’s more, I’d be willing to bet you’ve already thought of some use cases that you can implement in your business.
Here’s what is needed to get started sending texts from your IBM i:
- Get the phone number(s) of the recipient(s)
- Determine the cellphone carrier domain
- Build an RPG program to send the text message
Similarities to Email
Over the last several years, I’ve built many processes that send email notifications to customers and IT team members. I’ve also used email to send documents such as invoices or statements.
As you may know, native commands exist on IBM i, as well as in third-party packages that allow us to send emails. Recently, however, I was looking for a way to send text messages instead of emails as it seems most people prefer texts over emails these days.
My initial thought was to look for a web service I could consume to accomplish this task. After a short search, I found a few services I could use, but a cost was involved. Ideally, I’d like to be able to do this without incurring additional expense. After a little more digging and some testing, I found that it’s possible to send text messages from IBM i using native commands. As it turns out, these are the same commands I’ve been using to send emails! Using the SNDSMTPEMM (Send SMTP E-mail Message) command, I was able to send text messages easily.
It’s worth mentioning that you can also use the SNDDST (Send Distribution) command to send text messages, but for the purposes of this article, I’m using SNDSMTPEMM. I’m also making an assumption that you have already configured your system to send emails as I won’t be covering that here.
The Differentiator for Mobile
So, what’s the trick to sending text messages from IBM i? You need to know the mobile carrier and the gateway domain for the number you’re texting. For example, to send a plain text to a Verizon wireless customer, the address would be the phone number followed by the gateway domain—in this case, vtext.com. So, to send a text to a Verizon phone number, you would send it to 555-555-5555@vtext.com, for example. It’s important to note that if you’re sending a plain text message (a message with no attachments), it’s sent as a Short Message Service (SMS) message (see Figure 1).
If you want to send a text with an attachment, such as a jpg or pdf, you’ll send an Multimedia Message Service (MMS) message. I’m making the distinction because the gateway domain is different between SMS and MMS messages. I referred here (bit.ly/2vItzyw) to determine what gateway domain I needed for the examples referenced here.
Try it Yourself
Now that you have some background, let’s take a look at the sample program I created to send various types of text messages. I’ll start by showing how to send a simple text message (Figure 1) before moving on to sending a text with an attachment (Figures 2 and 3). As an added bonus, I’ll show how you can send a text that has a link to a website (Figure 4). The source code for this program is available on GitHub here.
Figure 1. Sending a plain text message
subject = 'This is a plain text message'; body = 'This is a plain text message'; recipient = '5555555555@vtext.com'; CmdStr = 'SNDSMTPEMM RCP(' + Quote + %trim(recipient) + Quote + ') ' + 'SUBJECT(' + Quote + %trim(subject) + Quote + ') ' + 'NOTE(' + Quote + %trim(body) + Quote + ')' + ' CONTENT(*HTML)'; Callp Run(Cmdstr:%Size(CmdStr)); Endsr;
If you’ve used the SNDSMTPEMM command before, this should look very familiar to you. The only difference between sending a text versus an email is the recipient to which you’re sending. In this example, I’m sending to a phone number (555-555-5555) and a gateway domain (vtext.com). Since I know this is a Verizon cell number, I know the gateway domain to use. If it had been a phone number for a different carrier, I’d use the link I provided to obtain that gateway domain. Assuming you have email functionality set up on your system, executing this command will send an SMS text message to the number provided.
That was pretty easy, right? Next, let’s take a look at how we can send a text with an attachment. I’ll show how to send a jpg, as well as a pdf. Each of the files I’m sending are stored in a folder in the IFS so I can access them easily.
Figure 2. Sending a text message with a jpg attachment
Begsr SendTextMessageJpg; subject = 'This text message has a jpg attachment'; body = 'This text message has a jpg attachment'; fileNameJpg = 'Nicole photo 1'; recipient = '5555555555@vzwpix.com'; filetype = '.jpg'; CmdStr = 'SNDSMTPEMM RCP(' + Quote + %trim(recipient) + Quote + ') ' + 'SUBJECT(' + Quote + %trim(subject) + Quote + ') ' + 'NOTE(' + Quote + %trim(body) + Quote + ')' + ' ATTACH((' + Quote + %trim(path) + '/' + %trim(fileNameJpg) + %trim(fileType) + Quote + ' *OCTET *BIN))' + ' CONTENT(*HTML)'; Callp Run(Cmdstr:%Size(CmdStr)); Endsr;
This is very similar to the way I sent a plain text message, but there are some small differences to point out. Notice that the gateway domain is slightly different (vzwpix.com vs. vtext.com). Although I’m sending the text to the same phone number, I need to use a gateway domain for an MMS message since I’m attaching a file.
In addition, I need to tell the SNDSMTPEMM command where to locate the attachment in the IFS. Finally, since I’m sending a ‘.jpg’ file, I need to specify the content type. There are many options to choose from (*MSG, *JPEG, *GIF, etc.), but I chose ‘*JPEG’ as that’s exactly what I’m sending.
Sending a text with a pdf as an attachment is very much the same as sending a jpg, except that I specify ‘*PDF’ as the content type for the attachment.
Figure 3. Sending a text message with a pdf attachment
Begsr SendTextMessagePdf; subject = 'This text message has a pdf attachment'; body = 'This text message has a pdf attachment'; fileNamePdf = '22H_-_Embedded_SQL_-_Beyond_The_Basics'; recipient = '5555555555@vzwpix.com'; filetype = '.pdf'; CmdStr = 'SNDSMTPEMM RCP(' + Quote + %trim(recipient) + Quote + ') ' + 'SUBJECT(' + Quote + %trim(subject) + Quote + ') ' + 'NOTE(' + Quote + %trim(body) + Quote + ')' + ' ATTACH((' + Quote + %trim(path) + '/' + %trim(fileNamePdf) + %trim(fileType) + Quote + ' *PDF))' + ' CONTENT(*HTML)'; Callp Run(Cmdstr:%Size(CmdStr)); Endsr;
The last example shows how to send a text with a link that will open a URL. The command is the same as sending a plain text, except that the body of the text contains a hyperlink.
Figure 4. Sending a text message with a URL
Begsr SendTextMessageWithLink; subject = 'Get the latest tips'; body = 'www.techchannel.com'; recipient = '5555555555@vtext.com'; CmdStr = 'SNDSMTPEMM RCP(' + Quote + %trim(recipient) + Quote + ') ' + 'SUBJECT(' + Quote + %trim(subject) + Quote + ') ' + 'NOTE(' + Quote + %trim(body) + Quote + ')' + ' CONTENT(*HTML)'; Callp Run(Cmdstr:%Size(CmdStr));
With a few pieces of information, sending text messages from IBM i can be accomplished easily. What’s more, it can be done using native functionality. And with the ability to send attachments and URL’s in your texts, you now have many more ways to communicate.
Get the Code
Want to try sending text messages from your IBM i? Find the source code on GitHub: bit.ly/2GR0mqM